22. Events and Delegates
There are a few, but important, Delegates exposed by AGX Dynamics for Unreal. These allow any user C++ code or Blueprint script to get callbacks when certain events occur.
22.1. Pre Step Forward
This Delegate is executed before each AGX Dynamics Step Forward call.
It passes one parameter, which is the current Simulation time.
The type of this parameter is float
.
To register a custom callback to this Delegate from C++, the following code can be used:
UAGX_Simulation* Simulation = UAGX_Simulation::GetFrom(this);
if (Simulation != nullptr)
Simulation->PreStepForward.AddDynamic(this, &UMyClass::MyFunction);
Notice that in this example, UMyClass::MyFunction must be a UFUNCTION taking one float
parameter.
Other variants of the Add function exists as well.
To see the full list, visit the Unreal Engine Documentation
To bind a Blueprint Event to this Delegate, the following can be done:
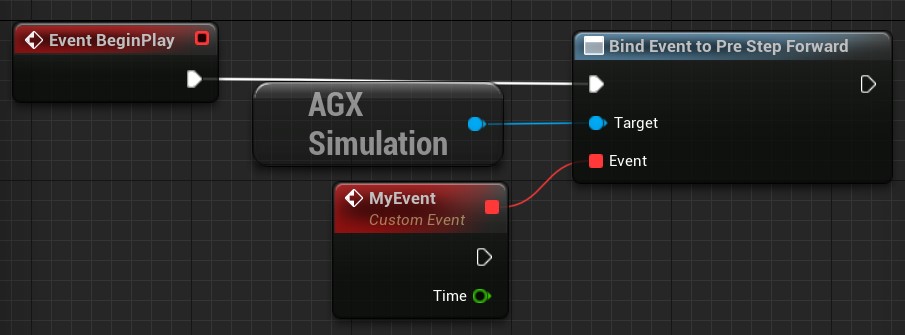
Binding a custom Event to the Pre Step Forward Delegate.
Note that the custom Event must take one input parameter of type float
.
22.2. Post Step Forward
This Delegate is executed after each AGX Dynamics Step Forward call.
It passes one parameter, which is the current Simulation time.
The type of this parameter is float
.
To register a custom callback to this Delegate from C++, the following code can be used:
UAGX_Simulation* Simulation = UAGX_Simulation::GetFrom(this);
if (Simulation != nullptr)
Simulation->PostStepForward.AddDynamic(this, &UMyClass::MyFunction);
Notice that in this example, UMyClass::MyFunction must be a UFUNCTION taking one float
parameter.
Other variants of the Add function exists as well.
To see the full list, visit the Unreal Engine Documentation
To bind a Blueprint Event to this Delegate, the following can be done:
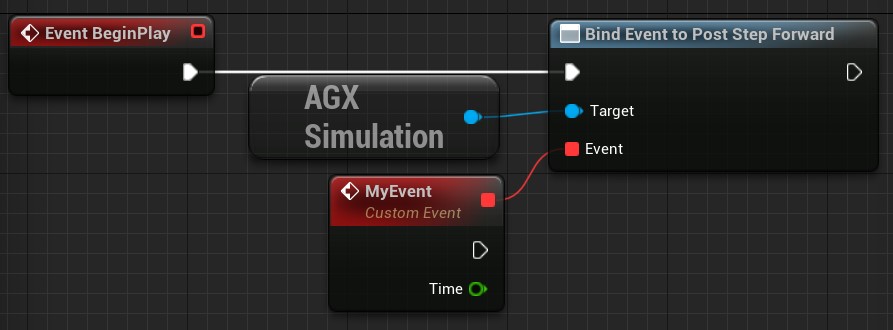
Binding a custom Event to the Post Step Forward Delegate.
Note that the custom Event must take one input parameter of type float
.